快速排序
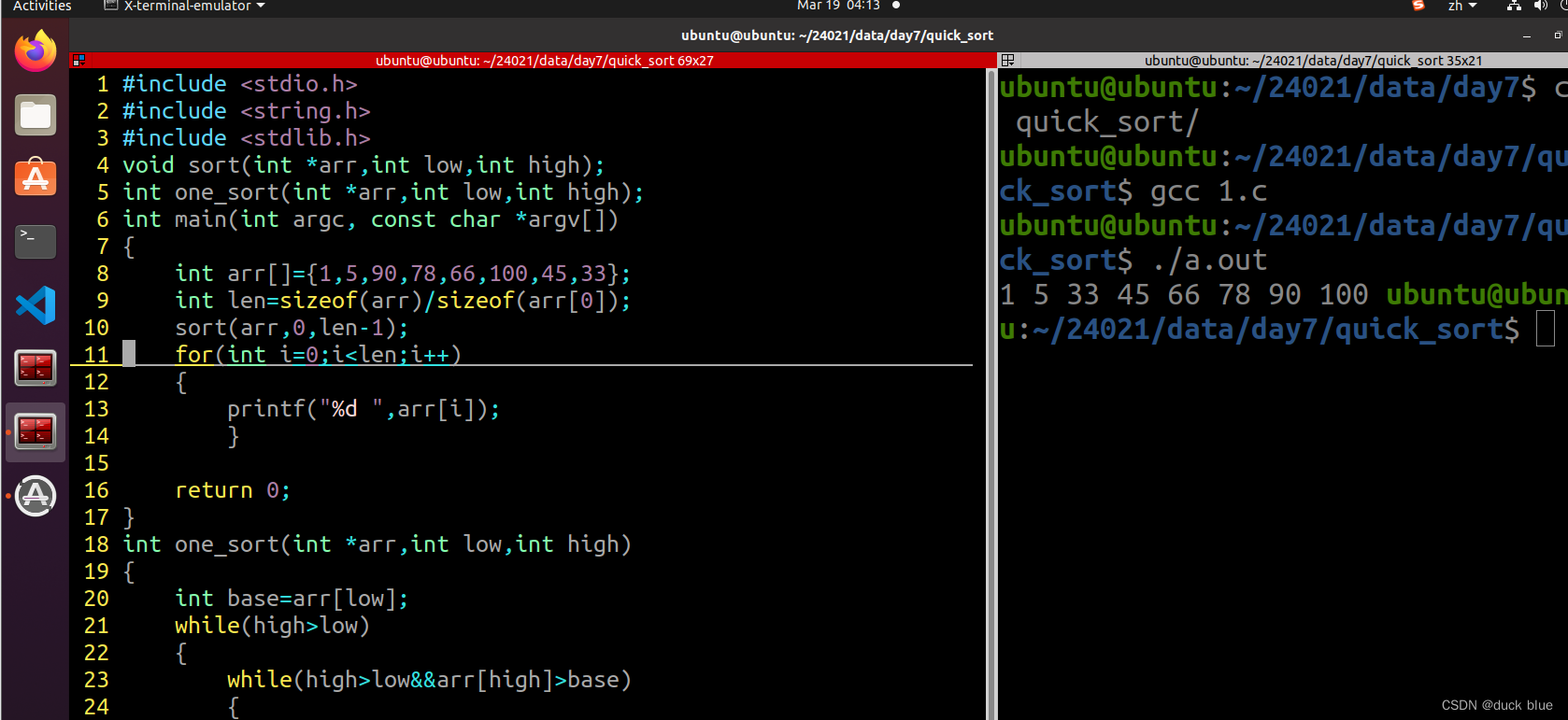
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
void sort(int *arr,int low,int high);
int one_sort(int *arr,int low,int high);
int main(int argc, const char *argv[])
{
int arr[]={1,5,90,78,66,100,45,33};
int len=sizeof(arr)/sizeof(arr[0]);
sort(arr,0,len-1);
for(int i=0;i<len;i++)
{
printf("%d ",arr[i]);
}
return 0;
}
int one_sort(int *arr,int low,int high)
{
int base=arr[low];
while(high>low)
{
while(high>low&&arr[high]>base)
{
high--;
}
arr[low]=arr[high];
while(high>low&&arr[low]<base)
{
low++;
}
arr[high]=arr[low];
}
arr[low]=base;
return low;
}
void sort(int *arr,int low,int high)
{
if(high>low)
{
int ret=one_sort(arr,low,high);
sort(arr,low,ret-1);
sort(arr,ret+1,high);
}
}
折半查找
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int half_search(int *arr,int low,int high,int k);
int main(int argc, const char *argv[])
{
int arr[]={12,34,54,67,88,99,100};
int len=sizeof(arr)/sizeof(arr[0]);
int k=88;
int num=half_search(arr,0,len-1,k);
printf("找到元素下标为%d\n",num);
return 0;
}
int half_search(int *arr,int low,int high,int k)
{
while(low<=high)
{
int mid=(low+high)/2;
if(arr[mid]==k)
{
return mid;
}
else if(arr[mid]>k){
high=mid-1;
}
else if(arr[mid]<k){
low=mid+1;
}
}
return -1;
}
哈希表查找
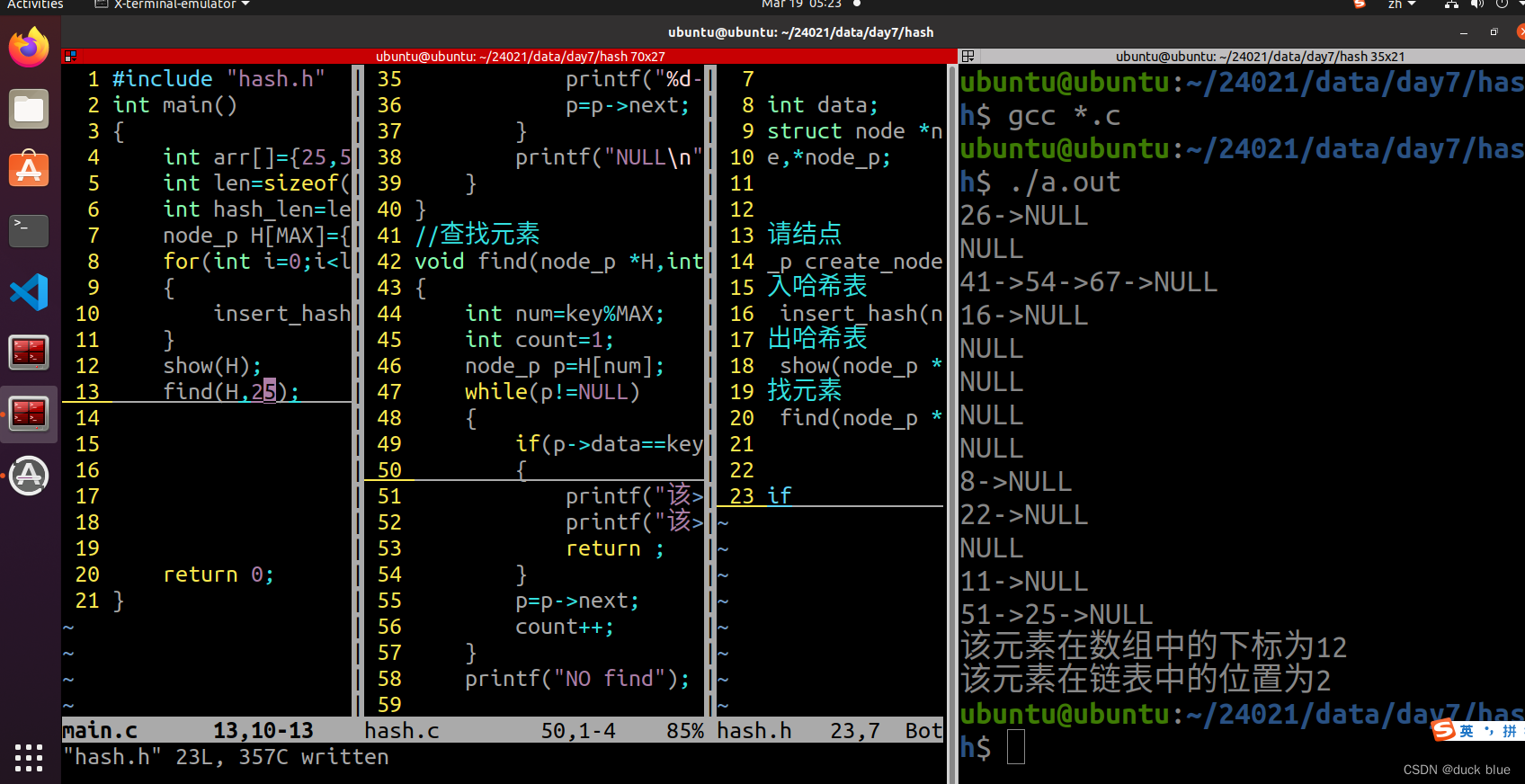
#include "hash.h"
int main()
{
int arr[]={25,51,8,22,26,67,11,16,54,41};
int len=sizeof(arr)/sizeof(arr[0]);
int hash_len=len*4/3;
node_p H[MAX]={0};
for(int i=0;i<len;i++)
{
insert_hash(H,arr[i]);
}
show(H);
find(H,25);
return 0;
}
#include "hash.h"
//申请结点
node_p create_node(int data)
{
node_p new=(node_p)malloc(sizeof(node));
if(new==NULL)
{
printf("申请空间失败");
return NULL;
}
new->data=data;
return new;
}
//存入哈希表
void insert_hash(node_p H[],int key)
{
int i=key%MAX;
node_p new=create_node(key);
new->next=H[i];
H[i]=new;
}
//输出哈希表
void show(node_p *H)
{
if(H==NULL)
{
printf("入参为空");
return ;
}
for(int i=0;i<MAX;i++)
{
node_p p=H[i];
while(p!=NULL)
{
printf("%d->",p->data);
p=p->next;
}
printf("NULL\n");
}
}
//查找元素
void find(node_p *H,int key)
{
int num=key%MAX;
int count=1;
node_p p=H[num];
while(p!=NULL)
{
if(p->data==key)
{
printf("该元素在数组中的下标为%d\n",num);
printf("该元素在链表中的位置为%d\n",count);
return ;
}
p=p->next;
count++;
}
printf("NO find");
}
#ifndef __HASH_H__
#define __HASH_H__
#include <stdio.h>
#include <stdlib.h>
#define MAX 13
typedef struct node
{
int data;
struct node *next;
}node,*node_p;
//申请结点
node_p create_node(int data);
//存入哈希表
void insert_hash(node_p H[],int key);
//输出哈希表
void show(node_p *H);
//查找元素
void find(node_p *H,int key);
#endif
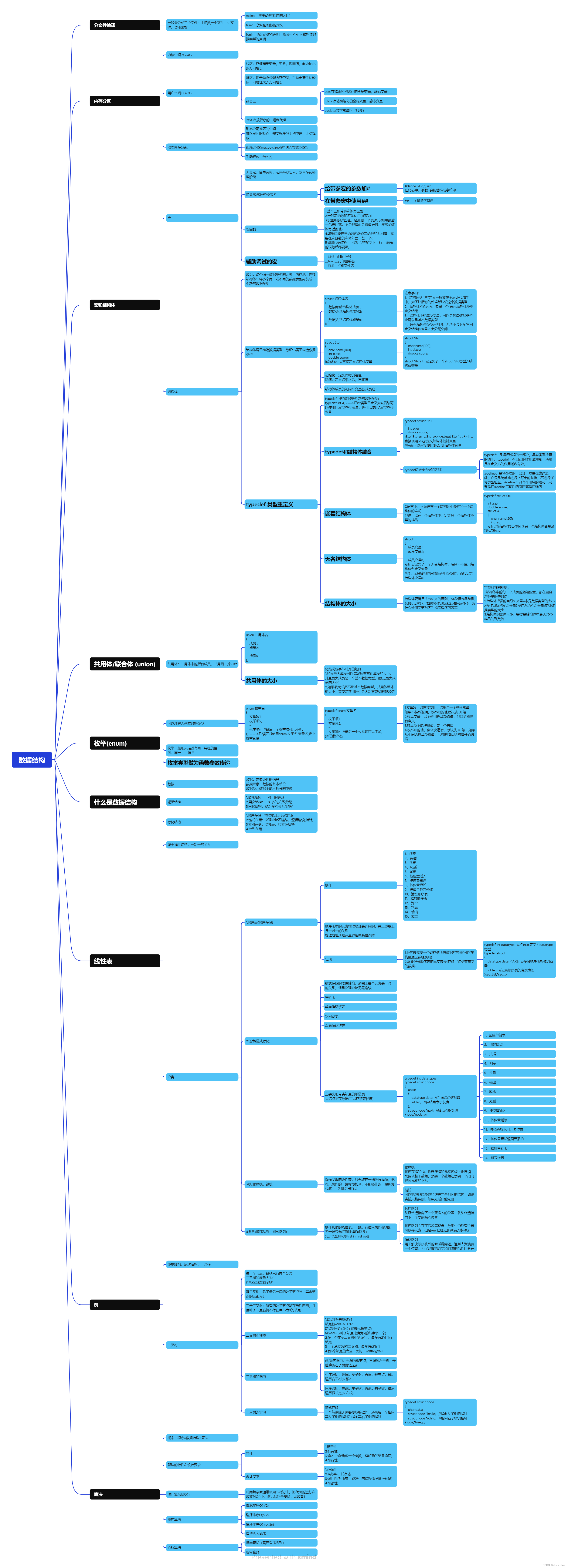