【二叉树】No. 0226 翻转二叉树【简单】👉力扣对应题目指路
希望对你有帮助呀!!💜💜 如有更好理解的思路,欢迎大家留言补充 ~ 一起加油叭 💦
欢迎关注、订阅专栏 【力扣详解】谢谢你的支持!
⭐ 题目描述:给你一棵二叉树的根节点 root ,翻转这棵二叉树,并返回其根节点。
- 示例: [4,2,7,1,3,6,9] -> [4,7,2,9,6,3,1]
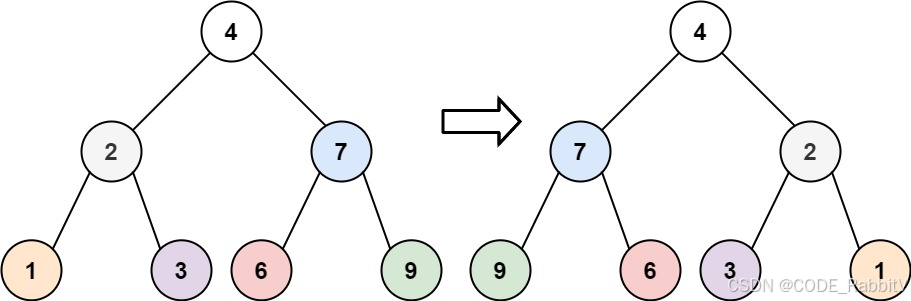
🔥 思路:由底向上(后序遍历),逐个翻转每一个子树
- 后续描述以由底向上(后序遍历)展开
- 由上向底(前序遍历) 也可,在代码中已注释标注
⭐题目准备之后续遍历:一定要先掌握后续遍历 ❗❗❗ 放在最后面啦,供不熟悉的友友参考~
参考如上思路,给出详细步骤如下:
- 步骤一⭐确定递归函数
traversal
参数及返回值
- 参数:仅需要获取当前需要翻转的根节点
current_node
- 返回值:不需要返回值
- 步骤二⭐确定递归终止条件:走到了
None
节点
- 说明当前下面没有待反转子树
- 步骤三⭐确定单层递归逻辑-后序处理:交换当前节点左右节点
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def invertTree(self, root: Optional[TreeNode]) -> Optional[TreeNode]:
def traversal(current_node): # ----------------- step 1
if current_node == None: # ----------------- step 2
return
# -- 前序处理的话,移动 step 3 到位置
traversal(current_node.left)
traversal(current_node.right)
# ------------------------------------------ step 3
temp = current_node.left
current_node.left = current_node.right
current_node.right = temp
traversal(root)
return root
⭐⭐⭐ 题目准备之后续遍历:一定要先掌握后续遍历 ❗❗❗
# Definition for a binary tree node.
# class TreeNode(object):
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def lowestCommonAncestor(self, root, p, q):
"""
:type root: TreeNode
:type p: TreeNode
:type q: TreeNode
:rtype: TreeNode
"""
self.result = []
def traversal(current):
if current == None:
return
print('-------------------------Hi, node: ', current.val)
traversal(current.left)
traversal(current.right)
print('----- current_val: ', current.val) // 观察此处的处理顺序,是后序
self.result.append(current.val)
traversal(root) ## self.result: [6, 7, 4, 2, 5, 0, 8, 1, 3]
- 对应的输出结果如下:
('-------------------------Hi, node: ', 3) ('-------------------------Hi, node: ', 5) ('-------------------------Hi, node: ', 6) ('----- current_val: ', 6) ('-------------------------Hi, node: ', 2) ('-------------------------Hi, node: ', 7) ('----- current_val: ', 7) ('-------------------------Hi, node: ', 4) ('----- current_val: ', 4) ('----- current_val: ', 2) ('----- current_val: ', 5) ('-------------------------Hi, node: ', 1) ('-------------------------Hi, node: ', 0) ('----- current_val: ', 0) ('-------------------------Hi, node: ', 8) ('----- current_val: ', 8) ('----- current_val: ', 1) ('----- current_val: ', 3)
希望对你有帮助呀!!💜💜 如有更好理解的思路,欢迎大家留言补充 ~ 一起加油叭 💦
欢迎关注、订阅专栏 【力扣详解】谢谢你的支持!
🔥 LeetCode 热题 HOT 100