1:使用 dup2 实现错误日志功能 使用 write 和 read 实现文件的拷贝功能,注意,代码中所有函数后面,紧跟perror输出错误信息,要求这些错误信息重定向到错误日志 err.txt 中去
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, const char *argv[])
{
//打开文件
int fd1=open("./task.txt",O_RDONLY);
int fd_err=open("./error.txt",O_WRONLY | O_CREAT | O_TRUNC,0664);
int fd2=open("./copy.txt",O_WRONLY | O_CREAT | O_TRUNC,0664);
if(fd_err==-1|fd1==-1|fd2==-1){
perror("fd_err:");
return -1;
}
//备份标准错误流
int res_err=dup(STDERR_FILENO);
//将标准错误流指针指向文件fd_err
dup2(fd_err,2);
//将文件fd1拷贝到文件fd2
while(1)
{
char buf[32]={0};
int res=read(fd1,buf,1);
if(res==0){break;}
write(fd2,buf,1);
}
//恢复stderr
dup2(res_err,2);
//关闭文件
close(fd1);
close(fd_err);
close(fd2);
return 0;
}
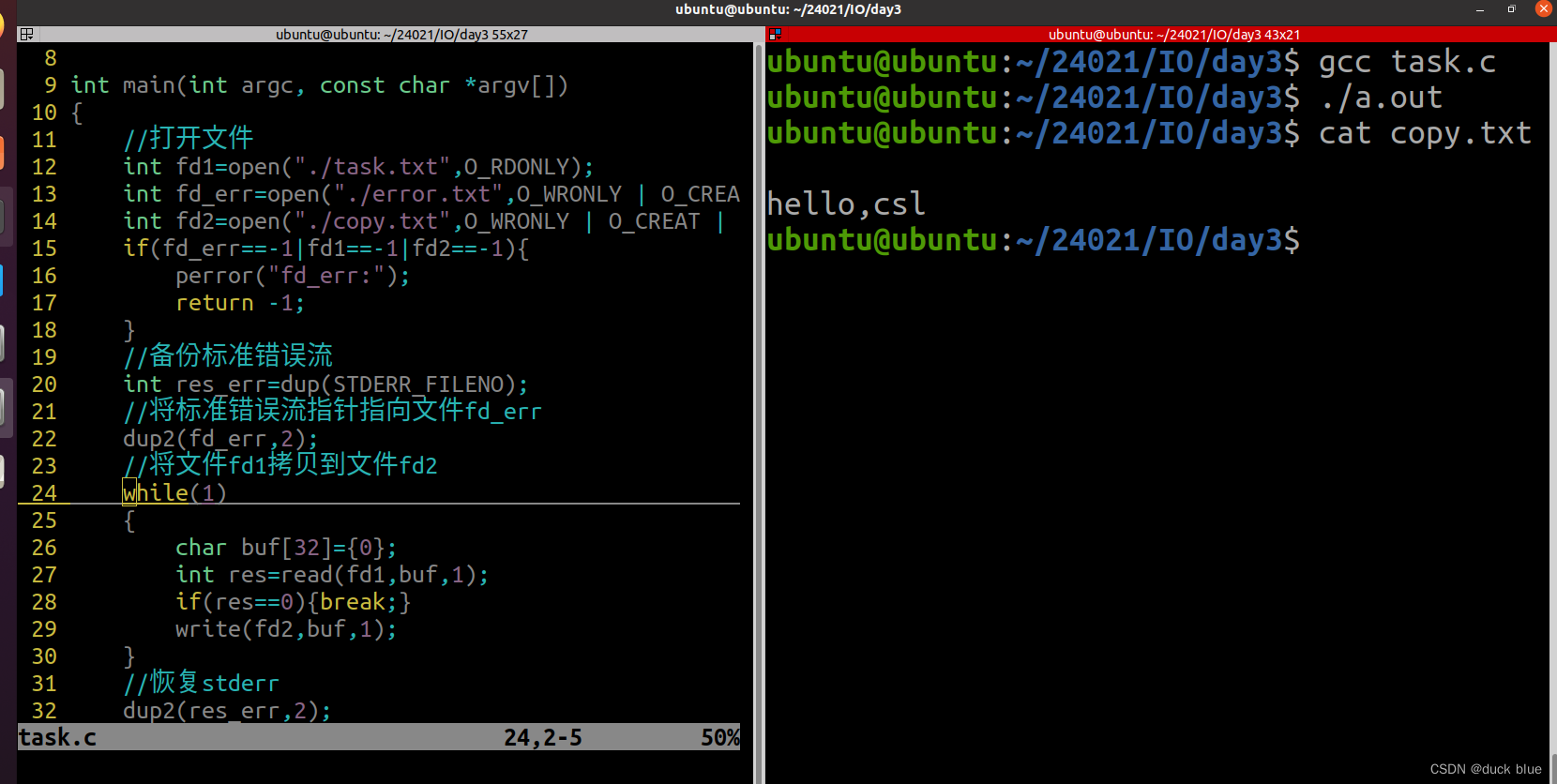
2:判断一个文件是否拥有用户可写权限,如果拥有,则去除用户可写权限,如果不拥有,则加上用户可写权限 权限更改函数:就是chmod函数,自己去man一下 要求每一次权限更改成功之后,立刻在终端显示当前文件的权限信息 :使用 ls -l显示(使用 system函数配合shell指令 ls -l 来实现)
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, const char *argv[])
{
struct stat buf;
int res=stat("./error.txt",&buf);
if(res==-1)
{
perror("error:");
return -1;
}
mode_t mode=buf.st_mode;
if((mode | S_IWUSR)==mode)
{
chmod("./error.txt",mode&(~S_IWUSR));
system("ls -lh ./hello.txt");
}
else
{
chmod("./error.txt",mode|S_IWUSR);
system("ls -lh ./hello.txt");
}
return 0;
}
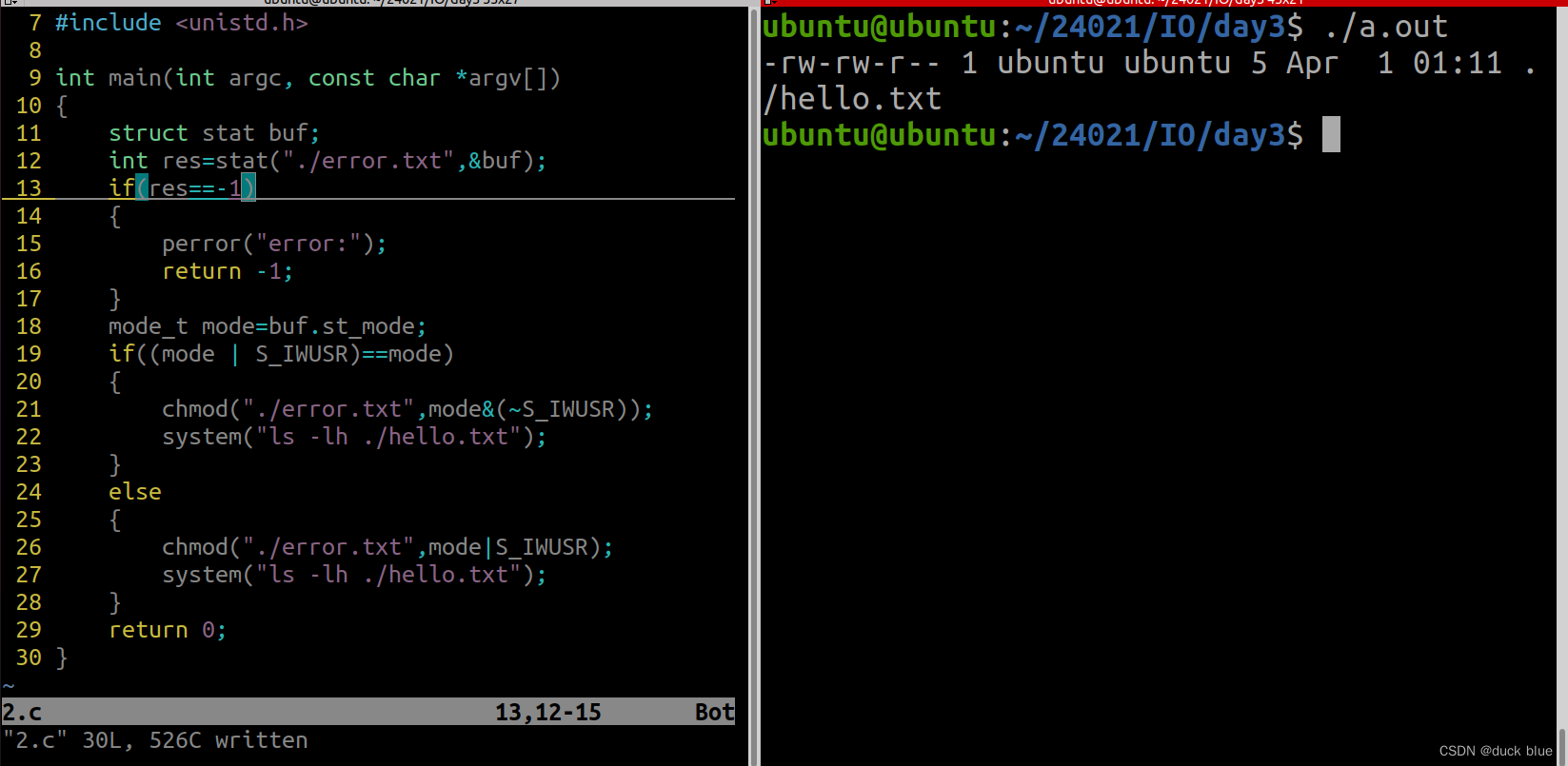