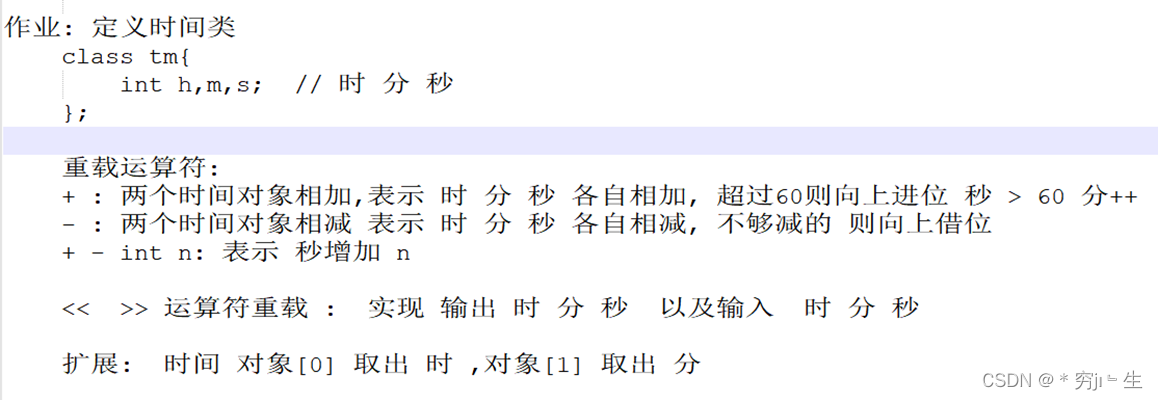
代码:
#include <iostream>
using namespace std;
// 定义时间类
class timee
{
private:
int h; // 时
int m; // 分
int s; // 秒
public:
// 无参构造
timee();
// 有参构造
timee(int h, int m, int s);
// 两个时间对象相加,表示时 分 秒各自相加,超过60 则向上进位
timee operator+(timee &t);
// 两个时间对象相减,表示时 分 秒各自相减,不够向上借位
timee operator-(timee &t);
// + - int n:表示秒增加n
timee operator+(int n);
timee operator-(int n);
// 扩展下标
int &operator[](int i);
void show();
};
timee::timee() : h(0), m(0), s(0) {}
timee::timee(int h, int m, int s)
{
this->h = h;
this->m = m;
this->s = s;
}
timee timee::operator+(timee &t)
{
timee temp;
temp.s = (s + t.s) % 60;
temp.m = (m + t.m + (s + t.s) / 60) % 60;
temp.h = (h + t.h + (m + t.m + (s + t.s) / 60) / 60);
return temp;
}
timee timee::operator-(timee &t)
{
timee temp;
temp.s = s - t.s;
if (temp.s < 0)
{
temp.m--;
temp.s += 60;
}
temp.m = temp.m + m - t.m;
if (temp.m < 0)
{
temp.h--;
temp.m += 60;
}
temp.h = temp.h + h - t.h;
if (temp.h < 0)
{
if (temp.s > 0)
{
temp.s = 60 - temp.s;
temp.m++;
}
if (temp.m > 0)
{
temp.m = 60 - temp.m;
temp.h++;
}
}
return temp;
}
timee timee::operator+(int n)
{
timee temp = *this;
temp.s += n;
temp.m += temp.s / 60;
temp.h += temp.m / 60;
temp.s %= 60;
temp.m %= 60;
return temp;
}
timee timee::operator-(int n)
{
timee temp = *this;
temp.s -= n;
temp.m -= (temp.s) / -60;
temp.h -= (temp.m) / -60;
temp.m %= -60;
temp.s %= -60;
if (temp.s < 0)
{
temp.m--;
temp.s += 60;
}
if (temp.m < 0)
{
temp.h--;
temp.m += 60;
}
return temp;
}
int &timee::operator[](int i)
{
switch (i)
{
case 0:
return s;
case 1:
return m;
case 2:
return h;
default:
cout << "超出范围" << endl;
return s;
}
}
void timee::show()
{
cout << h << "小时" << m << "分" << s << "秒" << endl;
}
int main(int argc, const char *argv[])
{
timee tm;
timee tm1(2, 20, 30);
timee tm2(5, 0, 0);
tm = tm1 + tm2;
tm.show();
tm = tm1 - tm2;
tm.show();
tm = tm1 - 80;
tm.show();
tm = tm2 + 90;
tm.show();
cout << "s = " << tm1[0] << " m = " << tm1[1] << " h = " << tm1[2] << endl;
return 0;
}
运行结果:
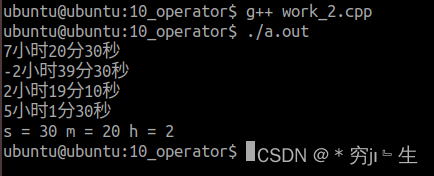