一、运行效果图
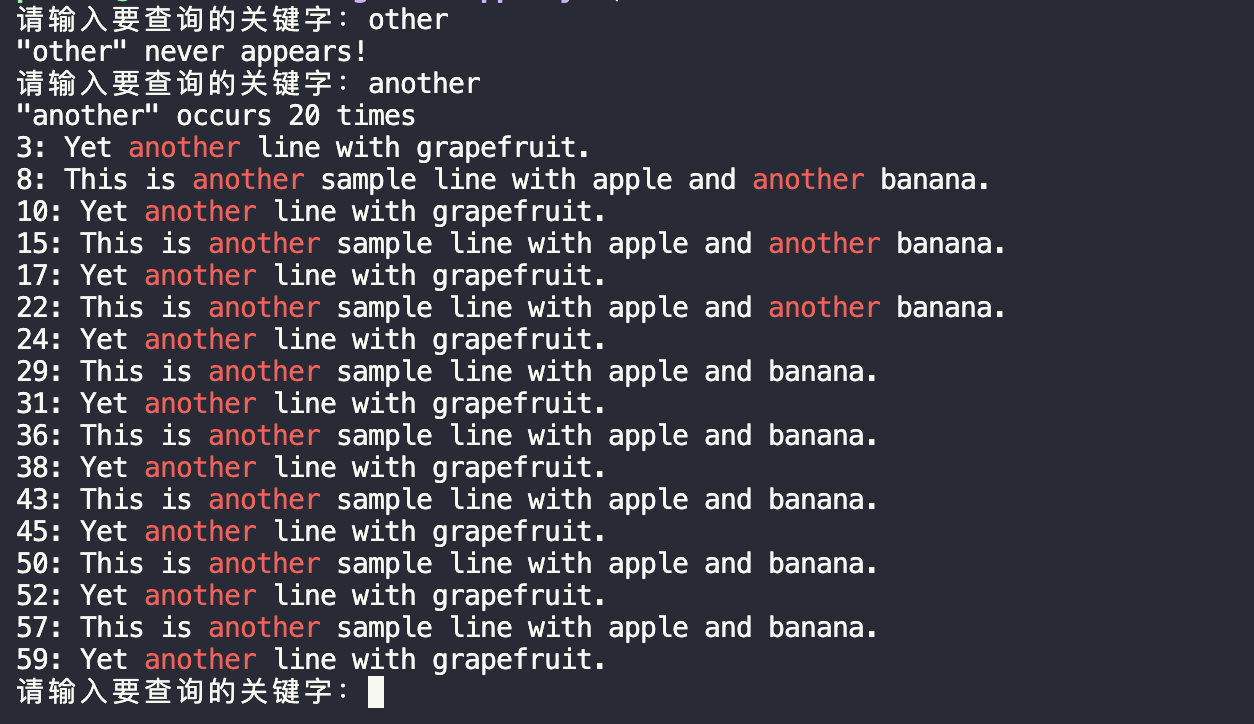
二、代码示例
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <map>
#include <set>
#include <vector>
#include <algorithm>
using namespace std;
class TextQuery {
public:
void readFile(const string &filename);
void query(const string &word);
private:
vector<string> _lines;
map<string, set<int>> _wordsNumbers;
map<string, int> _dict;
};
void TextQuery::readFile(const string &filename) {
ifstream ifs(filename);
if (!ifs.good()) {
ifs.close();
cerr << "open " << filename << " is fail" << endl;
return;
}
string line;
size_t lineNumber = 0;
while (getline(ifs, line)) {
++lineNumber;
_lines.push_back(line);
istringstream iss(line);
string word;
while (getline(iss, word, ' ')) {
word.erase(remove_if(word.begin(), word.end(), [](const char c) {
return !isalpha(c);
}),word.end());
if (word.size() == 0) {
continue;
}
++_dict[word];
auto it = _wordsNumbers.find(word);
if (it == _wordsNumbers.end()) {
set<int> numbers;
numbers.insert(lineNumber);
_wordsNumbers[word] = numbers;
} else {
it->second.insert(lineNumber);
}
}
}
ifs.close();
}
void TextQuery::query(const string &word) {
auto dictIt = _dict.find(word);
if (dictIt == _dict.end()) {
cout << "\"" << word << "\"" << " never appears!" << endl;
return;
}
cout << "\"" << word << "\"" << " occurs " << dictIt->second << ((dictIt->second > 1) ? " times" : " time") << endl;
auto wordsNumbersIt = _wordsNumbers.find(word);
for (const auto &lineNumber : wordsNumbersIt->second) {
cout << lineNumber << ": ";
istringstream iss(_lines[lineNumber - 1]);
string tempWord;
while (getline(iss, tempWord, ' ')) {
if (word == tempWord) {
cout << "\033[31m" << tempWord << "\033[0m" << " ";
} else {
cout << tempWord << " ";
}
}
cout << endl;
}
}
void test0() {
TextQuery tq;
tq.readFile("./test.txt");
string word;
while (cout << "请输入要查询的关键字:", getline(cin, word)) {
tq.query(word);
}
cout << endl;
}
int main(void) {
test0();
return 0;
}