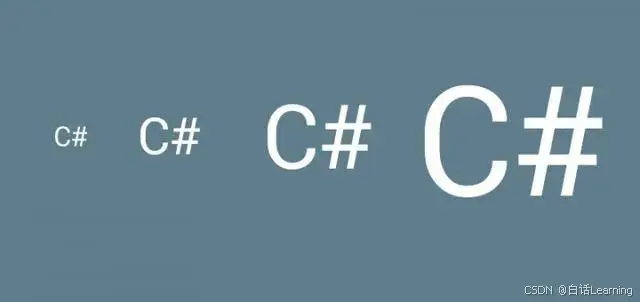
数据结构是计算机科学中非常重要的一部分,它帮助我们理解和操作数据。单链表是数据结构中的一种基本类型,它由一系列节点组成,每个节点包含数据域和指向列表中下一个节点的指针。在本文中,我们将详细讨论单链表的概念、操作和如何在几种常见的编程语言中实现它。
单链表的概念
单链表是一种线性数据结构,其中每个元素都是一个独立的对象,称为节点。每个节点都包含两个部分:数据域和指针域。数据域用于存储节点的数据,而指针域用于存储下一个节点的地址。
单链表可以分为两种类型:带头结点和不带头结点。带头结点的单链表在列表的第一个位置有一个特殊的头节点,它的数据域通常不存储有效数据,主要用于简化某些操作。不带头结点的单链表则直接从第一个有效节点开始。
单链表的特点是元素在内存中不是连续存储的,而是分散存储的。每个结点只存储指向下一个结点的指针,而不是存储整个列表的地址。因此,单链表中的元素在物理上是不连续的,它们通过指针连接起来形成一个逻辑上的线性序列。
单链表的操作
单链表的主要操作包括:
创建链表: 初始化一个空链表或带有特定数据的链表。
插入节点: 在链表的指定位置插入新节点。
删除节点: 根据节点的数据或位置删除节点。
遍历链表: 按照节点的顺序访问链表中的每个节点。
搜索节点: 根据节点的数据查找节点的位置。
释放链表: 删除链表中的所有节点,释放分配的内存。
单链表不带标准头结点示例
在单链表不带标准头结点的情况下,C语言、C#和C++的实现方式略有不同。下面分别给出每种语言的简单实现示例:
C语言实现
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
// 插入节点到链表尾部
void insertNode(struct Node** head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
struct Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
// 打印链表内容
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
// 测试主函数
int main() {
struct Node* head = NULL;
insertNode(&head, 1);
insertNode(&head, 2);
insertNode(&head, 3);
printf("Linked List: ");
printList(head);
return 0;
}
C#实现
using System;
public class Node {
public int data;
public Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
public class LinkedList {
private Node head;
// 插入节点到链表尾部
public void InsertNode(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
// 打印链表内容
public void PrintList() {
Node current = head;
while (current != null) {
Console.Write(current.data + " -> ");
current = current.next;
}
Console.WriteLine("NULL");
}
// 测试主函数
public static void Main() {
LinkedList list = new LinkedList();
list.InsertNode(1);
list.InsertNode(2);
list.InsertNode(3);
Console.Write("Linked List: ");
list.PrintList();
}
}
C++实现
#include <iostream>
using namespace std;
struct Node {
int data;
Node* next;
Node(int data) {
this->data = data;
this->next = nullptr;
}
};
// 插入节点到链表尾部
void insertNode(Node*& head, int data) {
Node* newNode = new Node(data);
if (head == nullptr) {
head = newNode;
return;
}
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
// 打印链表内容
void printList(Node* head) {
Node* current = head;
while (current != nullptr) {
cout << current->data << " -> ";
current = current->next;
}
cout << "NULL" << endl;
}
// 测试主函数
int main() {
Node* head = nullptr;
insertNode(head, 1);
insertNode(head, 2);
insertNode(head, 3);
cout << "Linked List: ";
printList(head);
return 0;
}
这些示例展示了如何在不带标准头结点的情况下实现单链表的基本操作(插入节点和打印链表)。在这种情况下,头指针需要作为参数传递到函数中,以便在链表为空时可以正确地修改头结点。
总结来说,单链表作为基础的数据结构,在很多编程问题中都有着广泛的应用,掌握好单链表的操作能力对理解和解决复杂的算法问题有很大帮助。